AES allows you to choose a 128-bit, 192-bit or 256-bit key, making it exponentially stronger than the 56-bit key of DES. AES is very fast and secure, and it is the de facto standard for symmetric encryption. Supported Python versions. # Both sides (encryptor and decryptor) must be in possession of the same secret key, # in order to communicate. API principles¶. Asymmetric keys are represented by Python objects. Each object can be either a private key or a public key (the method hasprivate can be used to distinguish them). A key object can be created in four ways: generate at the module level (e.g. Crypto.PublicKey.RSA.generate).The key is randomly created each time.
Update 2: 30-May-2016
There have been a number of comments and questions about whether this code is compliant or interoperable with other implementations. It is not. I wrote it as a non-crypto expert to teach myself more about AES. It was never intended to perform well or to be compatible with other AES implementations.

Update: 04-Apr-2009
I fixed two bugs in my AES implementation pointed out to me by Josiah Carlson. First, I was failing to pad properly files whose length was an even multiple of the block size. In those cases, bytes would be lost upon decrypting the file. Josiah also pointed out that I was using a static IV, which leaks information about messages which share common prefixes. This is a serious security bug and I was glad to have it pointed out.
Feel free to check out the changes I made or simply download the updated script.
I’ve put together a series of slides as well as a Python implementation of AES, the symmetric-key cryptosystem.
Source:pyAES.py
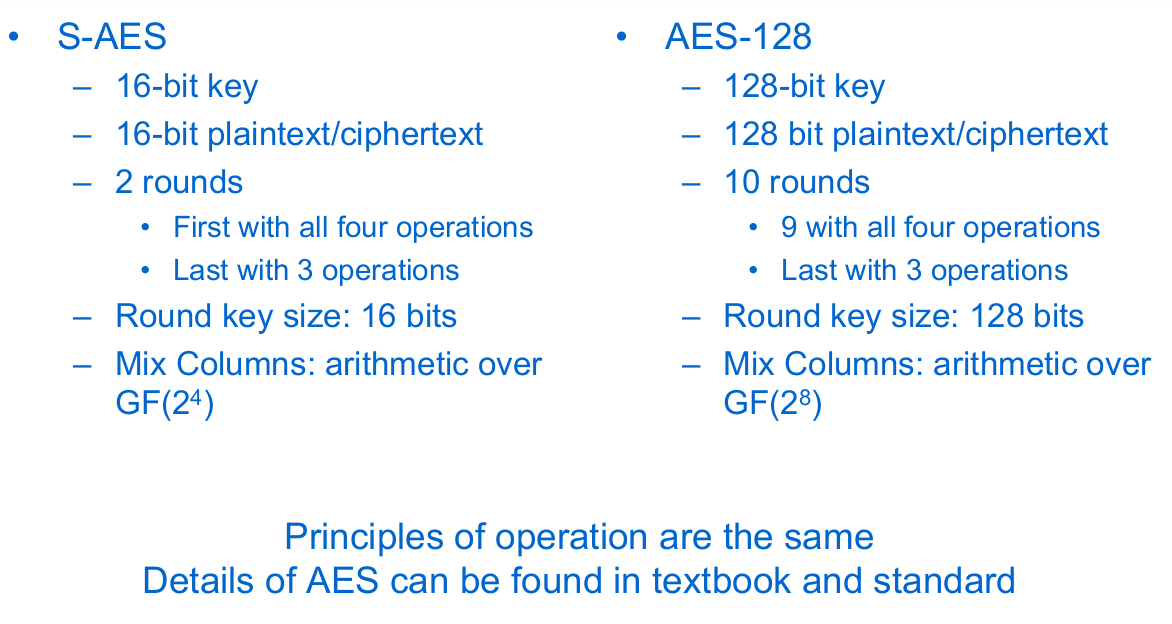
Sample Usage: (color added for clarity)
[brandon@zodiac pyAES]$ cat > testfile.txt
The sky was the color of television tuned to a dead channel.
[brandon@zodiac pyAES]$ ./pyAES.py -e testfile.txt -o testfile_encrypted.txt
Password:
Encrypting file: testfile.txt
Encryption complete.
[brandon@zodiac pyAES]$ ./pyAES.py -d testfile_encrypted.txt -o testfile_decrypted.txt
Password:
Decrypting file: testfile_encrypted.txt
Decryption complete.
[brandon@zodiac pyAES]$ cat testfile_decrypted.txt
The sky was the color of television tuned to a dead channel.
[brandon@zodiac pyAES]$ md5sum *
19725cef7495fd55540728759a6262c8 pyAES.py
2fffc9072a7c09f4f97862c0bceb6021 testfile_decrypted.txt
3e57070eaf1b4adf7f43b38e1c5ee631 testfile_encrypted.txt
2fffc9072a7c09f4f97862c0bceb6021 testfile.txt
Symmetric Key Cryptography
- Identical keys used to encrypt/decrypt messages
- Can be implemented as block ciphers or stream ciphers
Strengths:
- Speed
- Much less computationally intensive than public-key crypto
- Easy to implement in hardware as well as software
Weaknesses:
- Key Management
- n users require n(n-1)/2 keys for all to communicate
- secure key distribution is a challenge
- Cannot be used (directly) for authentication or non-repudiation
Python Crypto Cipher Aes
AES – The Advanced Encryption Standard
- Rijndael algorithm invented by Joan Daemen and Vincent Rijmen and selected as AES winner by NIST in 2001
- AES uses fixed block size of 128-bits and key sizes of 128, 192 or 256 bits (though Rijndael specification allows for variable block and key sizes)
- Most of the calculations in AES are performed within a finite field
- There are a finite number of elements within the field and all operations on those elements result in an element also contained in the field
AES Operations
- AES operates on a 4×4 matrix referred to as the state
- 16 bytes 128 bits block size
- All operations in a round of AES are invertible
- AddRoundKey – each byte of the round key is combined with the corresponding byte in the state using XOR
- SubBytes – each byte in the state is replaced with a different byte according to the S-Box lookup table
- ShiftRows – each row in the state table is shifted by a varying number of bytes
- MixColumns – each column in the state table is multiplied with a fixed polynomial
AES Operation – AddRoundKey
- Each byte of the round key is XORed with the corresponding byte in the state table
- Inverse operation is identical since XOR a second time returns the original values
AES Operation – SubBytes
- Each byte of the state table is substituted with the value in the S-Box whose index is the value of the state table byte
- Provides non-linearity (algorithm not equal to the sum of its parts)
- Inverse operation is performed using the inverted S-Box
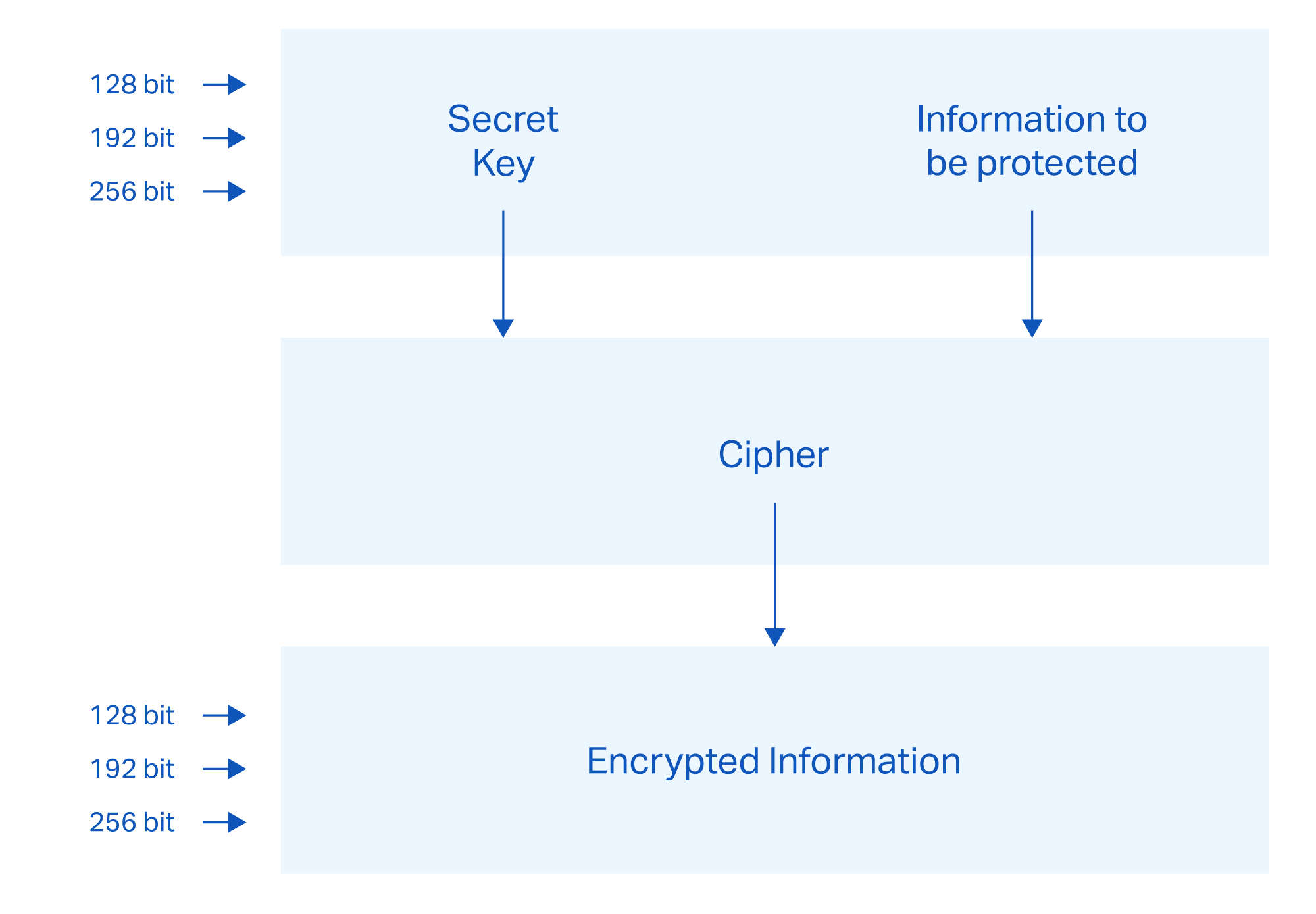
AES Operation – ShiftRows
- Each row in the state table is shifted left by the number of bytes represented by the row number
- Inverse operation simply shifts each row to the right by the number of bytes as the row number
AES Operation – MixColumns
- MixColumns is performed by multiplying each column (within the Galois finite field) by the following matrix:
- The inverse operation is performed by multiplying each column by the following inverse matrix:
AES – Pulling It All Together

The AES Cipher operates using a varying number of rounds, based on the size of the cipher key.
Python Aes Encryption Code
- A round of AES consists of the four operations performed in succession: AddRoundKey, SubBytes, ShiftRows, and MixColumns (MixColumns is omitted in the final round)
- 128-bit key → rounds, 192-bit key → 12 rounds, 256-bit key → 14 rounds
- The AES cipher key is expanded according to the Rijndael key schedule and a different part of the expanded key is used for each round of AES
- The expanded key will be of length (block size * num rounds+1)
- 128-bit cipher key expands to 176-byte key
- 192-bit cipher key expands to 208-byte key
- 256-bit cipher key expands to 240-byte key
AES – Key Expansion Operations
AES key expansion consists of several primitive operations:
- Rotate – takes a 4-byte word and rotates everything one byte to the left, e.g. rotate([1,2,3,4]) → [2, 3, 4, 1]
- SubBytes – each byte of a word is substituted with the value in the S-Box whose index is the value of the original byte
- Rcon – the first byte of a word is XORed with the round constant. Each value of the Rcon table is a member of the Rinjdael finite field.
AES – Key Expansion Algorithm (256-bit)
Pseudo-code for AES Key Expansion:
- expandedKey[0:32] → cipherKey[0:32] # copy first 32 bytes of cipher key to expanded key
- i → 1 # Rcon iterator
- temp = byte[4] # 4-byte container for temp storage
- while size(expandedKey) < 240
temp → last 4 bytes of expandedKey# every 32 bytes apply core schedule to temp
if size(expandedKey)%32 0
temp = keyScheduleCore(temp, i)
i → i + 1
# since 256-bit key -> add an extra sbox transformation to each new byte
for j in range(4):
temp[j] = sbox[temp[j]]
# XOR temp with the 4-byte block 32 bytes before the end of the current expanded key.
# These 4 bytes become the next bytes in the expanded key
expandedKey.append( temp XOR expandedKey[size(expandedKey)-32:size(expandedKey)-28]
Another function to note…
AES – Encrypting a Single Block
- state → block of plaintext # 16 bytes of plaintext are copied into the state
- expandedKey = expandKey(cipherKey) # create 240-bytes of key material to be used as round keys
- roundNum → 0 # counter for which round number we are in
- roundKey → createRoundKey(expandedKey, roundNum)
- addRoundKey(state, roundKey) # each byte of state is XORed with the present roundKey
- while roundNum < 14 # 14 rounds in AES-256
roundKey → createRoundKey(expandedKey, roundNum)
# round of AES consists of 1. subBytes, 2. shiftRows, 3. mixColumns, and 4. addRoundKey
aesRound(state, roundKey)
roundNum → roundNum + 1 - # for the last round leave out the mixColumns operation
roundKey = createRoundKey(expandedKey, roundNum)
subBytes(state)
shiftRows(state)
addRoundKey(state) - return state as block of ciphertext
